Xamarin的基本控件及常见用法
· 阅读需 6 分钟
Xamarin 是一个开放源代码平台,用于通过 .NET 构建适用于 iOS、Android 和 Windows 的新式高性能应用程序。本文记录了Xamarin框架的基本控件,并给出了相关例子。
提示
2023.01更新:咳,这玩意现在用的人少了,国内用的就更少了,至少我是不推荐再学了。搞跨平台还是Qt、React Native或者Flutter。有选择权的话,试试 渐进式 Web 应用(Progressive Web Apps, WPA) 也不错嘛。
一一介绍
一些通用的属性
Margin 边距
简而言之,就是设置控件距左上右下的边距,注意,不是HTML里的上右下左!
VerticalTextAlignment & HorizontalTextAlignment 垂直/水平居中
意思很明显,就是定义控件的文本的对齐方式,有 Center
, Strat
, End
三个选项,分别对应:居中、左对齐、右对齐。
FontSize 字体大小
不多说,设置文本字体的大小
以Label控件为例
<Label Margin="8"
VerticalTextAlignment="center"
HorizontalTextAlignmant="Center"
FontSize="24"
Text="Apple"
x:Name="AppleLabel"/>
表示一个上下左右边距均为8,垂直/水平居中,字体24号,显示文本"Apple
",名称为"AppleLabel
"的Label控件。
提示
加个浏览器窗口是因为图和背景颜色边界不明显,实际运行没有哈。
另外,左上角的黑条条是调试的时候用的,实际运行不会显示。
http://localhost:3000
Label 文本框
最简单的控件,用于显示文本,常见属性有:
<Label Text="显示的文本"
VerticalTextAlignment="center"
TextColor="Red"
x:Name="AppleLabel"
FontSize="24"/>
http://localhost:3000
Button 按钮
按钮,按了就可以除非指定事件,比如:
<Button x:Name="MyPopupButton"
Text="Click me!"
Margin="8"
Clicked="MyPopupButton_Clicked"/>
配合如下代码:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="Blog.MainPage">
<StackLayout>
<Label Text="显示的文本"
VerticalTextAlignment="center"
TextColor="Red"
x:Name="AppleLabel"
FontSize="24"/>
<Button x:Name="MyPopupButton"
Text="Click me!"
Margin="8"
Clicked="MyPopupButton_Clicked"/>
</StackLayout>
</ContentPage>
using System;
using Xamarin.Forms;
namespace Blog
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private void MyPopupButton_Clicked(object sender, EventArgs e)
{
AppleLabel.Text = "Apple";
}
}
}
效果:
http://localhost:3000
Grid 表
一般用于排版,可以把其他控件放入其中,常见用法:
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition width="Auto"/>
<ColumnDefinition width="*"/>
</Grid.ColumnDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition width="Auto"/>
<ColumnDefinition width="Auto"/>
<ColumnDefinition width="Auto"/>
</Grid.ColumnDefinitions>
<!-- Something others -->
</Grid>
这个示例创建了一个3行2列的表,示例在本文末尾
ScrollView 滚动视图
顾名思义,就是鼠标滚轮可以控制的可滚动视图
<ScrollView HeightRequest="150"/>
最好要定义它的高度
用Label实现的超链接
通过附加属性使Label可点击,并添加下划线
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="Blog.MainPage">
<Grid>
<Label Grid.Row="4"
Grid.Column="1"
Text="Click me!"
x:Name="ClickMeButton"
Margin="8"
TextDecorations="Underline">
<Label.GestureRecognizers>
<TapGestureRecognizer Tapped="ClickMeHyperlinkButton_Tapped"/>
</Label.GestureRecognizers>
</Label>
</Grid>
</ContentPage>
配合C#代码:
using System;
using Xamarin.Essentials;
using Xamarin.Forms;
namespace HelloWorld
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
//注意要异步打开
private async void ClickMeHyperlinkButton_Tapped(object sender,
EventArgs e)
{
await Browser.OpenAsync("https://www.bilibili.com");
}
}
}
Switch 开关
没什么好说的
<Switch Margin="8"/>
Entry 输入框
同样没什么可说的
<Entry Margin="4"/>
完整的例子
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="Blog.MainPage">
<StackLayout>
<ScrollView>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Text="Grid:"
Grid.Row="0"/>
<Grid Grid.Row="0"
Grid.Column="1"
Margin="8">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="2*"/>
<ColumnDefinition Width="3*"/>
<ColumnDefinition Width="1*"/>
</Grid.ColumnDefinitions>
<Label Text="hello 1234 5678"
Margin="4"
FontSize="24"
Grid.ColumnSpan="3"/>
<Label Text="用户名:"
Grid.Row="1"
Grid.Column="0"
VerticalTextAlignment="Center"/>
<Entry Grid.Row="1"
Grid.Column="1"
Margin="4"/>
<Button Text="确定"
Grid.Row="2"
Grid.Column="1"
Margin="4"/>
</Grid>
<Label Text="StackLayout:"
Grid.Row="1"
Grid.Column="0"/>
<StackLayout Orientation="Horizontal"
Grid.Row="1"
Grid.Column="1"
Margin="8"
Spacing="0">
<BoxView Color="Blue"
WidthRequest="50"
HeightRequest="50" />
<StackLayout Orientation="Vertical"
Spacing="0">
<BoxView Color="Red"
WidthRequest="50"
HeightRequest="25" />
<BoxView Color="Yellow"
WidthRequest="50"
HeightRequest="25" />
</StackLayout>
</StackLayout>
<Label Text="ScrollViewr:"
Grid.Row="2"
Grid.Column="0"/>
<ScrollView Grid.Row="2"
Grid.Column="1"
HeightRequest="150">
<StackLayout>
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
<Label Text="123" />
</StackLayout>
</ScrollView>
<Label Grid.Row="4"
Grid.Column="0"
Text="超链接"/>
<Label Grid.Row="4"
Grid.Column="1"
Text="Click me!"
x:Name="ClickMeButton"
Margin="8"
TextDecorations="Underline">
<Label.GestureRecognizers>
<TapGestureRecognizer Tapped="ClickMeHyperlinkButton_Tapped"/>
</Label.GestureRecognizers>
</Label>
<Label Grid.Row="5"
Grid.Column="0"
Text="弹窗:"/>
<Button x:Name="MyPopupButton"
Text="Click me!"
Grid.Row="5"
Grid.Column="1"
Margin="8"
Clicked="MyPopupButton_Clicked"/>
<Label Grid.Row="6"
Grid.Column="0"
Text="开关:"/>
<Switch Grid.Row="6"
Grid.Column="1"
Margin="8"/>
<Label Text="滑块"
Grid.Row="7"
Grid.Column="0"/>
<StackLayout Orientation="Horizontal"
Grid.Row="7"
Grid.Column="1"
Margin="8">
<Slider x:Name="MySlider"
WidthRequest="200"
Maximum="100"
Minimum="0"
HorizontalOptions="Start"/>
<Label Text="{Binding Value, Source={x:Reference MySlider}}"
FontSize="24"
Margin="4,0,0,0"/>
</StackLayout>
</Grid>
</ScrollView>
</StackLayout>
</ContentPage>
using System;
using Xamarin.Essentials;
using Xamarin.Forms;
namespace Blog
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private void MyPopupButton_Clicked(object sender, EventArgs e)
{
DisplayAlert("提示", "这是一个简单的弹窗", "确定");
}
private async void ClickMeHyperlinkButton_Tapped(object sender, EventArgs e)
{
await Browser.OpenAsync("https://www.bilibili.com");
}
}
}
http://localhost:3000
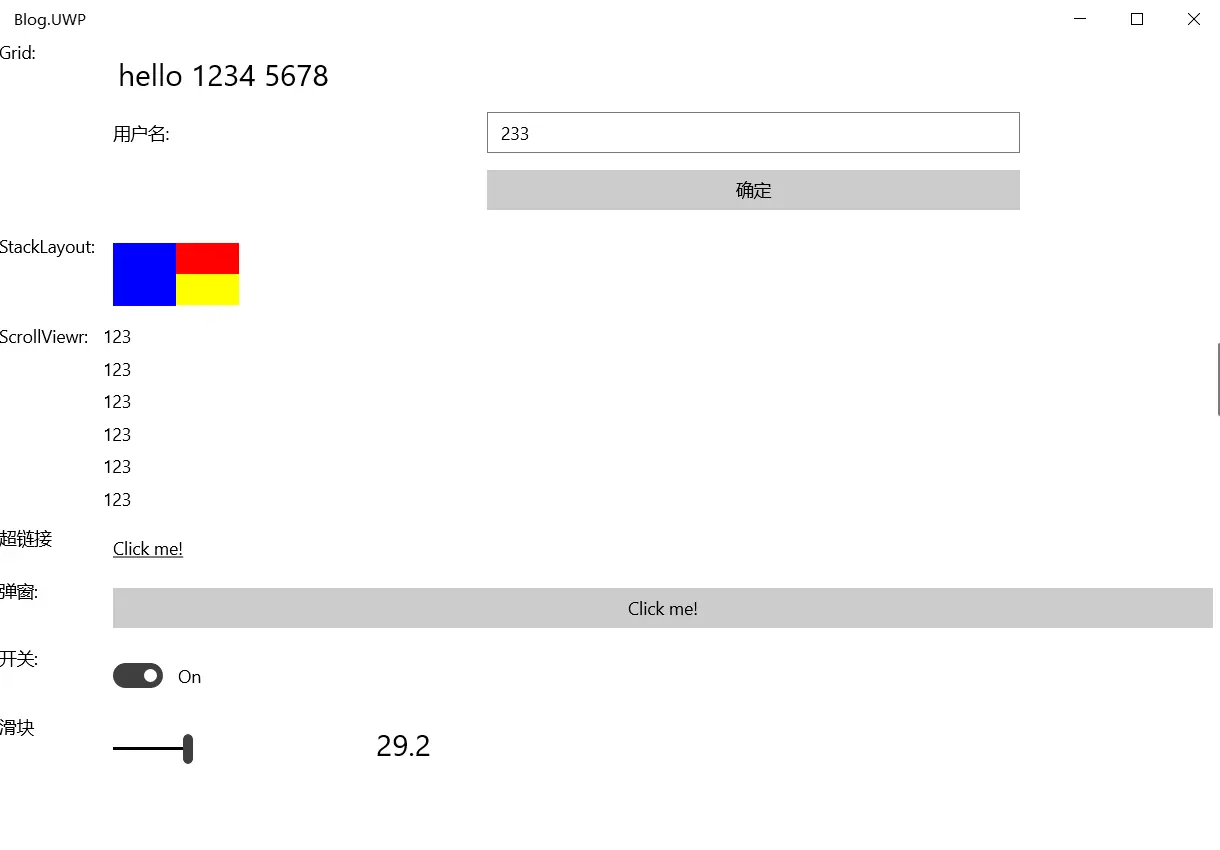